주소창에 http://.../list?path=d:\root 와 같이 path를 자신의 local pc의 dir로 지정하면 파일의 리스트를 출력..
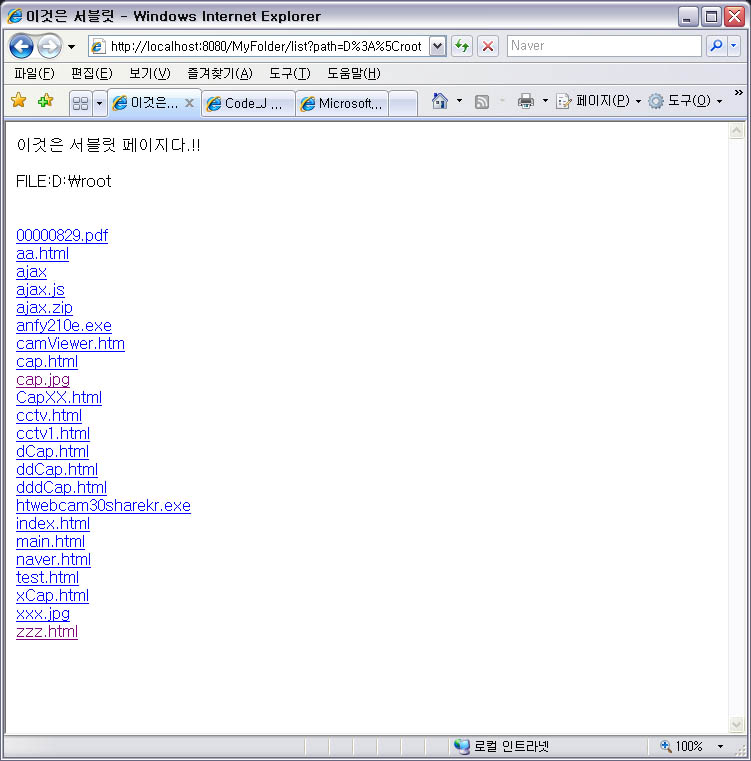
디렉토리는 해당 디렉토리 내부로 이동..
파일 전송을 위한 서블릿 파일
--------------------------------
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.net.*;
import java.io.*;
/**
* Servlet implementation class for Servlet: sender
*
*/
public class sender extends javax.servlet.http.HttpServlet implements
javax.servlet.Servlet {
static final long serialVersionUID = 1L;
/*
* (non-Java-doc)
*
* @see javax.servlet.http.HttpServlet#HttpServlet()
*/
public sender() {
super();
}
/*
* (non-Java-doc)
*
* @see javax.servlet.http.HttpServlet#doGet(HttpServletRequest request,
* HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String filePath = request.getParameter("filePath");
filePath = new String(filePath.getBytes("8859_1"), "euc-kr");
System.out.println("--->" + filePath);
File file = new File(filePath);
if(file.exists())
{
response.setContentType(this.getMimeType(filePath));
response.setBufferSize(2048);
response.setContentLength((int)file.length());
OutputStream os = response.getOutputStream();
byte[] buf = new byte[2048];
InputStream is = new FileInputStream(file);
int n = -1;
while((n = is.read(buf)) > 0)
{
os.write(buf, 0, n);
}
is.close();
os.flush();
os.close();
}
response.encodeRedirectURL("list?path=" + file.getParent());
}
public String getMimeType(String fileUrl)
throws java.io.IOException, MalformedURLException {
String type = null;
URL u = new File(fileUrl).toURL();
URLConnection uc = null;
uc = u.openConnection();
type = uc.getContentType();
return type;
}
/*
* (non-Java-doc)
*
* @see javax.servlet.http.HttpServlet#doPost(HttpServletRequest request,
* HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
}
파일 리스트 출력을 위한 서블릿파일
----------------------------------------------------------
package service.file;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.net.*;
/**
* Servlet implementation class for Servlet: list
*
*/
public class list extends javax.servlet.http.HttpServlet implements
javax.servlet.Servlet {
static final long serialVersionUID = 1L;
/*
* (non-Java-doc)
*
* @see javax.servlet.http.HttpServlet#HttpServlet()
*/
public list() {
super();
}
/*
* (non-Java-doc)
*
* @see javax.servlet.http.HttpServlet#doGet(HttpServletRequest request,
* HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
String rootDir = (String) request.getParameter("path");
//rootDir = URLDecoder.decode(rootDir, "utf-8");
rootDir = new String(rootDir.getBytes("8859_1"), "euc-kr");
response.setContentType("text/html;charset=euc-kr");
System.out.println(">" + rootDir);
PrintWriter pw = response.getWriter();
pw.print("<html><head><title>이것은 서블릿</title></head><body>"
+ "이것은 서블릿 페이지다.!!<br><br>FILE:" + rootDir + "<br><br>");
File dir = new File(rootDir);
if (dir.exists() && dir.isDirectory())
{
pw.print("<br>");
for (File file : dir.listFiles())
{
if (file.isDirectory())
{
pw.print("<a href=list?path=" + URLEncoder.encode(file.getCanonicalPath()
,"euc-kr")+ ">" + file.getName() + "</a><br>");
} else if (file.isFile()) {
pw.print("<a href=sender?filePath=" + URLEncoder.encode(file.getCanonicalPath()
,"euc-kr") + ">" + file.getName() + "</a><br>");
}
}
}
pw.print("</body></html>");
pw.flush();
pw.close();
}
/*
* (non-Java-doc)
*
* @see javax.servlet.http.HttpServlet#doPost(HttpServletRequest request,
* HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
}
'Expired > Java Works' 카테고리의 다른 글
NIO 강좌 - 3 (0) | 2008.02.09 |
---|---|
NIO 강좌 - 2 (0) | 2008.02.09 |
Java로 EXE 파일 실행..(Excuting exe file by Java Program) (0) | 2007.12.29 |
실시간 블로그 이미지 갱신.. (0) | 2007.12.02 |
[JMF] JPEG Capture by USB Cam (0) | 2007.11.28 |